The most important Java commands in an overview
Using the right Java commands, you can write programs faster and easier, making Java work for you. We’ve collected a set of the most important Java commands so you can learn how to include them in your work.
- 99.9% uptime
- PHP 8.3 with JIT compiler
- SSL, DDoS protection, and backups
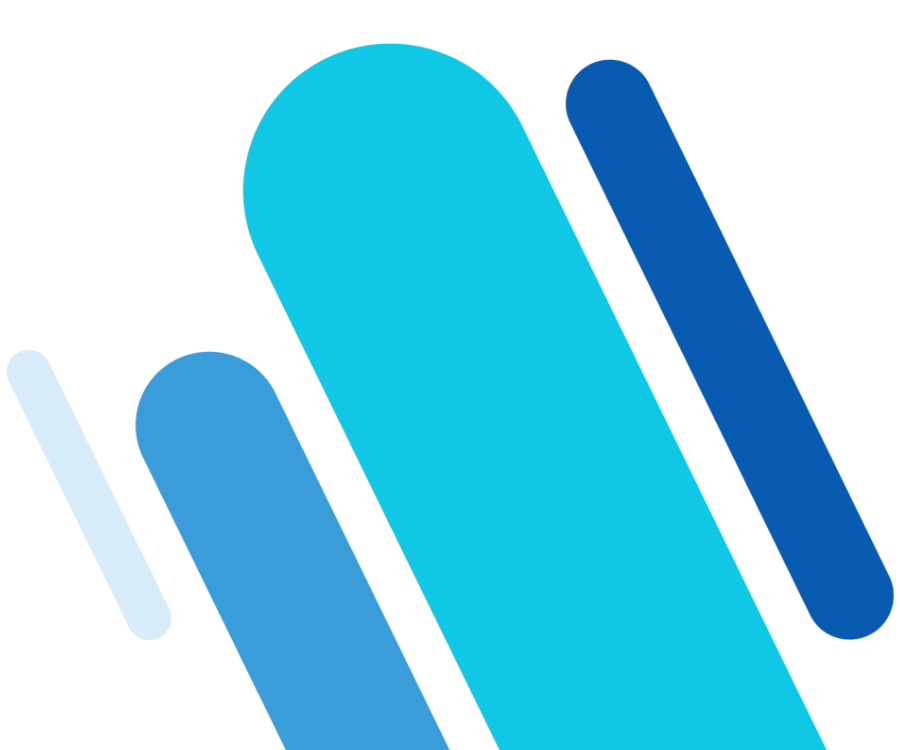
What are Java commands?
Anyone who’s come across programming will know that Java is a programming language. It works with fixed syntax and can execute programs by using fixed steps in the source code. Besides Java operators, the different Java commands are among the most important building blocks of the language. In the following, you will find a list of the most important Java commands that you can use when programming.
Basic Java commands
You will need to know the basic Java commands at the beginning when you want to start writing your own program. Additionally, these Java commands also help you in your daily work on your program after it has gone through its teething troubles, and are therefore important to look at first.
Java commands | Description |
---|---|
java -version | Use this command to determine which version of Java is installed on your device. |
javac -version | Use javac -version to determine the Java compiler version. |
whereis | whereis is your main search tool in Java. Use this command to find components within a directory. |
main | This command is your starting point and is used to declare or instantiate new classes. |
class | Through class you define methods and properties of an object. |
public | Through public, other classes can also access the program. |
object | With object you assign an object to a variable. |
static | With static you ensure that changes of the variable of an object are also taken over for the other objects of a class. |
Important output commands in Java
Java has several output commands. These include:
Java commands | Description |
---|---|
void | void indicates that no data is to be passed and the corresponding location is left empty instead. |
args | args is used to pass values in the form of an array from the command line to the Java main method. |
string | string defines a string in Java. |
System.out.println | With this command you have a text output via the console. After the output, a newline is also inserted. |
private | private ensures that a function is only executed within the respective class. |
int (integer) | int specifies integers in Java. These can be positive or negative. |
int firstnumber; | This command creates an int variable and gives it the name “firstnumber”. |
double | double specifies decimal numbers and stores data with double precision. |
double result; | This command creates a double variable and gives it the name “result”. |
char | char is used to specify exactly one character. |
char onecharacter; | This command creates a char variable and gives it the name “onecharacter”. |
try catch | try returns a chain of commands that you want to execute. catch is a backup that performs a second operation if the first one doesn’t work. |
if ( condition ) {First statement}else {Second statement} | With if and else you can check if a variable has a certain value. If it meets the condition, the first statement is executed. If the condition does not apply, the second statement takes effect. |
Different loops in Java
In Java, you have the option of using loops to repeatedly perform certain operations. This works via a Boolean operator. As long as a termination condition remains “true” (i.e. valid), the application continues to execute. If this is no longer true and the condition is “false” (i.e. untrue), the program exits the loop.
Java commands | Description |
---|---|
while ( termination condition ) {statement;} | As long as the termination condition is true, the statement is executed again and again. If the condition no longer applies, the program is terminated. |
do {statement;} while ( termination condition ) | In contrast to while, do-while is a foot-controlled loop. This means that the statement is executed first and the program only then checks whether the termination condition is “true” or “false”. |
for ( int i = 1;i <= 5;i++ {System.out.println} | Also with for, a statement is executed as long as the termination condition is “true”. In contrast to the while loop, however, for has a run variable that can be used to determine in advance how often the statement is to be repeated. |
Java commands for user input
To deal with input from users, one of the things that helps is the use of the following Java commands:
Java commands | Description |
---|---|
BufferedReader input=newBufferedReader (new InputStreamReader (System.ini)); | This command allows you to read in user input. |
strInput = input.readLine ( ); | With this command the user input is read in and stored in the variable “strInput”. |
Java commands for advanced Java users
If you already have experience creating programs in Java, these advanced Java commands can give you additional useful options.
Java commands | Description |
---|---|
extends | Using this command, you ensure that a new class inherits all the features of the base class. |
return | Assign a return value to a method. |
super keyword | Using super keyword, a superclass (or superclass) will inherit all attributes and methods to all subclasses. |
this | The “this” command acts as reference variables in Java and allow you to access the current object in the program. |
Java commands for working with the Graphical User Interface (GUI)
When using the Java GUI, there are some additional helpful Java commands besides the ones already listed:
Java commands | Description |
---|---|
Test = jTextfield.getText( ); | With this command you can view an input from the text field “jTextfield” and save it in the previously set variable “Test”. |
jLabel.setText ( Test ); | This command plays out the contents of the “Test” variable on the “jLabel” label. |
double box ( double value ) { | If you need to use a function more frequently in a program, it makes things easier if you create a method for it, as in this example. Afterwards you simply access the method and this then contains the entire code. |
squarenumber= square(2); | By this command the respective method gets the value “2” as a parameter. The variable “squarenumber” then stores the return value of this method. |
How are Java commands built in?
The structure of Java commands follows a similar pattern. Each command ends with a “;”. Here’s an example of a simple command:
public class Main {
public static void main ( String [ ] args {
System.out.println ( "Here is your sample sentence" );
}
}
The command can be found at this location: “System.out.println ( "Here is your sample sentence" );”. It causes the text inside the two quotes to be printed to the console.