How to use Python dictionary
The Python dictionary is an important data type in Python’s internet programming language. It is used to link terms and meanings together. Key-value pairs are used to create tables, data collections, or inventories. The Python dictionary can also be used with Python “for” loops, Python “if else” statements, and Python “while” loops.
- DNS management
- Easy SSL admin
- API documentation
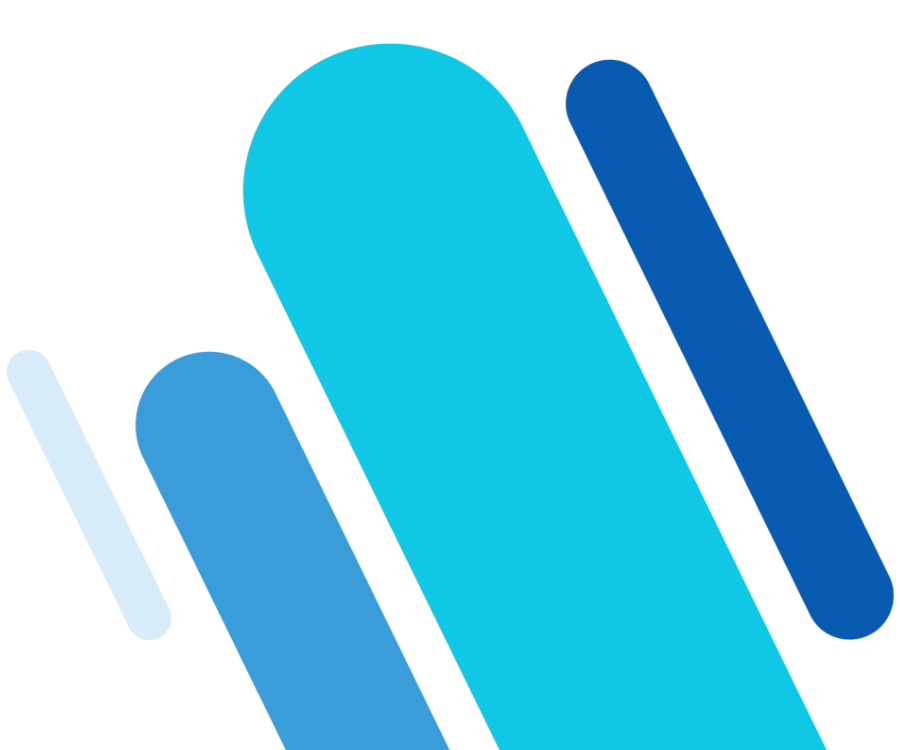
What is a Python dictionary?
A Python dictionary is a data type which links terms and meanings. This structure allows keys and values to be linked, stored, and later retrieved. Similar to a regular dictionary where a term is associated with an explanation or translation, the Python dictionary creates an association table which cannot contain duplicates. This means that each key can only occur once.
The structure of a Python dictionary
A Python dictionary is always in curly braces. The key and its corresponding value are connected by a colon, and the resulting pairs are separated by commas. Theoretically, a Python dictionary may contain any number of key-value pairs. The key is enclosed in quotation marks. You probably already know how to write it from our Python tutorial. See the example below:
ages = {'Jim': 42, 'Jack': 13, 'John': 69}
The Python dictionary is read by placing the correct key in square braces. Below you will find how it is written in code:
age_jim = ages['Jim']
assert age_jim == 42
Python dictionary example
The Python dictionary’s structure and functionality can be explained with a simple example. Countries and capitals are assigned and outputted in the following structure. The Python dictionary should look like this:
capitals = { "UK": "London", "FR": "Paris", "DE": "Berlin" }
Use the square braces to query a specific element:
capitals["FR"]
assert capitals["FR"] == 'Paris'
The output would be the value associated with the key “France”, which would be “Paris”.
Alternative structure
You can also build a Python dictionary without content and fill it afterwards. An empty Python dictionary is always created first for this and it should look like this:
capitals = { }
Complete the Dictionary:
capitals["UK"] = "London"
capitals["FR"] = "Paris"
capitals["DE"] = "Berlin"
When you query the Python dictionary “capitals”, the following will be outputted:
{ "UK": "London", "FR": "Paris", "DE": "Berlin" }
How do I change the Python dictionary?
You can also modify your Python dictionary later on. Simply write the following to attach key-value pairs:
capitals[ "IT" ] = "Roma"
print(capitals)
It should now look like this:
{ "UK": "London", "FR": "Paris", "DE": "Berlin", "IT": "Roma" }
Use the following code to change a value within a key-value pair:
capitals = { "UK": "London", "FR": "Paris", "DE": "Berlin", "IT": "???" }
assert capitals["IT"] == "???"
capitals["IT"] = "Roma"
assert capitals["IT"] == "Roma"
The output then reads:
{ "UK": "London", "FR": "Paris", "DE": "Berlin", "IT": "Roma" }
How do I remove key-value pairs from the Python dictionary?
There are three options available to subsequently remove a key-value pair from the Python dictionary: del, pop, and popitem.
Remove with del
A simple inventory is a good example of the del method. Let’s assume that a bakery offers 100 rolls, 25 loaves of bread, and 20 croissants when it opens for business. The corresponding Python dictionary would look like this:
stock = { "rolls": 100, "breads": 25, "croissants": 20 }
If the bakery has sold all its croissants and wants to delete them from its listing, the del command can be used to do this:
del stock["croissants"]
print(stock)
You must make sure that you always use the del command in conjunction with a key-value pair with this method. Otherwise, the entire Python dictionary will be deleted. This is a Python problem which occurs relatively often.
Remove with pop
The second way to remove key-value pairs from a Python dictionary is pop. This stores the removed value in a new variable. It works as follows:
marbles = { "red": 13, "green": 19, "blue": 7, "yellow": 21}
red_marbles = marbles.pop("red")
print(f"We've removed {red_marbles} red marbles.")
print(f"Remaining marbles are: {marbles}")
The output will look like this:
We've removed 13 red marbles.
Remaining marbles are: {'green': 19, 'blue': 7, 'yellow': 21}
Remove with popitem
Use popitem to remove the last key-value pair from your Python dictionary. This is the code:
last = marbles.popitem()
print(last)
print(marbles)
Your output will look like this:
('yellow', 21)
{ "One": 1, "Two": 2, "Three": 3 }
Other methods for the Python dictionary
There are a few other methods that work with a Python dictionary. Below is a list of the most important options:
Method | Description |
---|---|
Clear | Clear removes all key-value pairs from the Python dictionary. |
Copy | Copy creates a copy of the dictionary in storage. |
Get | Get determines the value by entering the key. |
Keys | Keys reads the keys of your dictionary. |
Update | Update extends a Python dictionary by one. Duplicate keys are overwritten in the attached dictionary. |
Values | Values queries all your dictionary’s values. |