How to pause programs with Python sleep
Python sleep is a function which allows you to pause a program momentarily and resume it afterwards without any changes. This simple command in the internet programming language allows you to time outputs, display the time, initiate a countdown, or set an alarm.
- DNS management
- Easy SSL admin
- API documentation
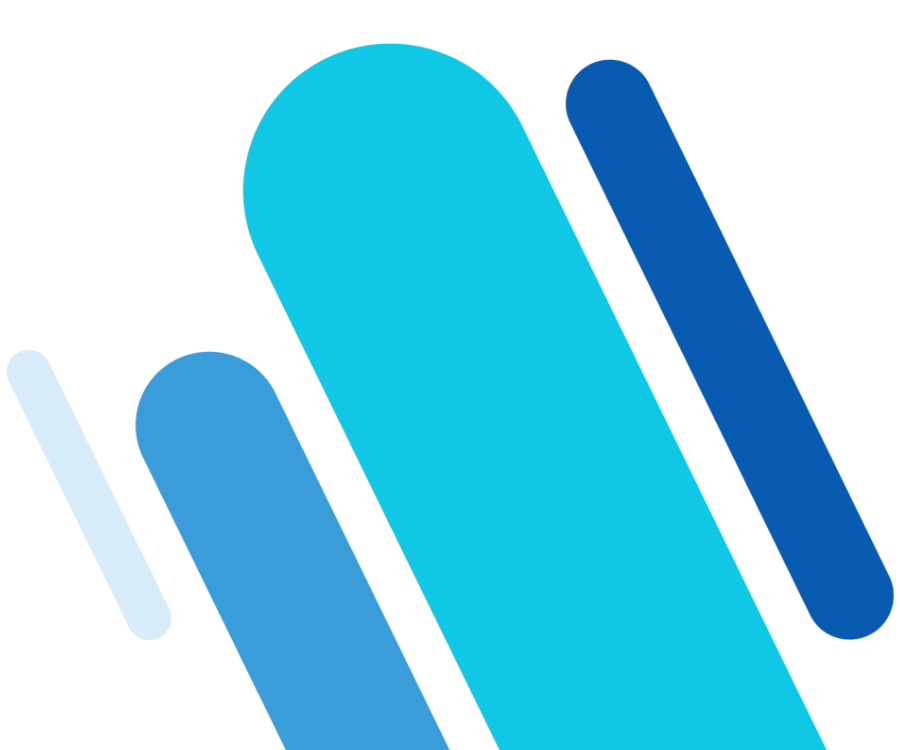
What is Python sleep?
Python sleep gives you the option to pause your program for a set amount of time and then automatically resume running it after the waiting period has ended. This may give users time to read through an instruction or create their own digital counter. Python sleep can be used in conjunction with the time module. While the structure and use of sleep is relatively simple when used in conjunction with Python if else statement, Python while loops, or Python for loops, the function enables some pretty complicated operations. Apart from the scheduled delay, sleep does not interfere with the program, unlike Python break and continue.
What is syntax and functionality of Python sleep?
The syntax of Python sleep always looks like this:
time.sleep(t)
t indicates the waiting time and is usually specified in seconds.
Python sleep usually pauses a specified sequence of events. A command is given first, followed by a break with a specified time window, and a statement for the action that should be performed after the break is given at the end.
A simple example illustrates this perfectly. One line is output in this example. After that, the program pauses for five seconds and then outputs a second line. The code is:
import time
print("This sentence will be output immediately.")
time.sleep(5)
print("This sentence will be output five seconds later.")
Alternatively, assign the value x for the time.sleep to create a pause. The code will look like this for a pause of exactly one minute:
import time
wait_time = 60
time.sleep(wait_time)
You can also specify the waiting time in milliseconds. Simply divide the input by 1,000 to do this. It should look like this:
import time
print("This sentence will be output immediately.")
time.sleep(500/1000)
print("This sentence will be output five hundred milliseconds later.")
How do I create a digital clock with Python sleep?
You can create a digital clock with Python sleep using a traditional while loop, which allows you to use the function in a whole new way. The while loop is covered early in most Python tutorials. Simply request the current time and output it in the format hour, minutes, seconds. Then set a delay of exactly one second and command the program to loop again using Python sleep. Continuing the loop without an end time creates an accurate digital clock in just a few steps. This is the code:
import time
while True:
localtime = time.localtime()
result = time.strftime("%I : %M : %S %p", localtime)
print(result)
time.sleep(1)
The output should be written using the 12-hour clock and looks like this:
04 : 16 : 47 PM
04 : 16 : 48 PM
04 : 16 : 49 PM
04 : 16 : 50 PM
04 : 16 : 51 PM
The time entry will just keep running continuously if there is no end point set. To end the endless loop, press the key combination [Ctrl] + [C].
How do I create a timer?
Python sleep is also excellent as a timer. Follow these steps to set it to five minutes:
import time
print("Here we go : %s" % time.ctime())
time.sleep(300)
print("Now the time is up : %s" % time.ctime())
The output will look like this:
Here we go : Wed Dec 14 11:10:14 2022
Now the time is up : Wed Dec 14 11:15:14 2022
There are exactly 300 seconds, or five minutes, between the two outputs.
How do I countdown with Python sleep?
You can also use the Python sleep function to count in reverse. In the following example, a countdown from 10 to 0 will be created. The principle is similar to the last example, but a for loop is used this time.
import time
for i in reversed(range (1, 11)):
print(i)
time.sleep(1)
print("Here we go!")
The program will count the numbers in descending order from 10. Python sleep reveals a new value every second. Once the loop has run to 1, the message “Here we go!” appears instead of 0.
Python sleep and multithreading
Multithreading is also possible with Python sleep and it involves the execution of several simultaneous processes. The next example shows two outputs being requested with two different threads using for loops. Python sleep lets you control the execution of the instruction which brings a fixed order to the program. It works like this:
import threading
import time
def first():
for i in range(5):
time.sleep(0.5)
print("This is the first function")
def second():
for i in range(5):
time.sleep(0.7 )
print("This is the second function")
t1 = threading.Thread(target = first)
t2 = threading.Thread(target = second)
t1.start()
t2.start()