How to use PHP classes for object-oriented programming
PHP classes structure code into independent logical modules, each of which has specific properties and methods. This promotes reusability and the management of large projects.
What are PHP classes?
PHP classes are like templates or blueprints that are used to create objects. A class defines the properties (variables or attributes) and methods (functions) that an object will have. Classes are an essential part of object-oriented programming and can be used in conjunction with other PHP functions, for example. You can also create classes to use PHP to retrieve information from a MySQL database.
By using Deploy Now from IONOS, you benefit from a state-of-the-art hosting solution for your applications. Deploy Now offers first-class reliability and a wide range of powerful automation functions for optimum support for your projects.
How to define PHP classes
To define a class in PHP, we use the keyword class, followed by the class name and curly brackets. In between, we write the properties and methods of the class.
class Name {
// php class example properties and methods
}
phpHere’s a more concrete example:
class Animal {
// Properties
public $name;
public $color;
// Methods
function set_name($name) {
$this->name = $name;
}
function get_name() {
return $this->name;
}
}
phpThe “Animal” class has the two properties “name” and “color” as well as two methods for setting (set_name) and retrieving (get_name) the name.
To learn more about PHP programming, take a look at the PHP tutorial from our guide. We’ve compared the advantages and disadvantages of PHP vs. Python and PHP vs. JavaScript for you.
- DNS management
- Easy SSL admin
- API documentation
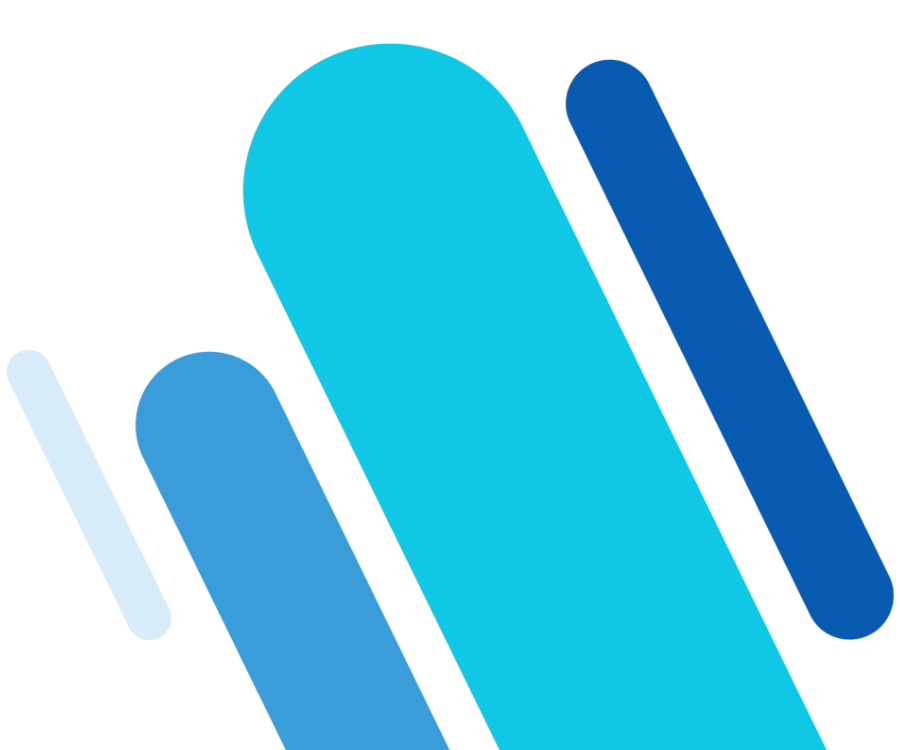
What is the connection between PHP classes and objects?
Objects are instances of a class. When you create an instance of a class, you get a specific object that can use the properties and methods defined in that class. You create objects from a class using the new keyword.
class Animal {
// Properties
public $name;
public $color;
// Methods
function set_name($name) {
$this->name = $name;
}
function get_name() {
return $this->name;
}
}
$dog = new Animal();
$cat = new Animal();
$dog->set_name('Tom');
$cat->set_name('Mickey');
echo $dog->get_name();
echo "<br>";
echo $cat->get_name();
phpHere, “dog” and “cat” are instances of the “Animal” class, which we give their own names.
Furthermore, PHP classes can be inherited, which means you can derive from an existing class to define a new class. The derived class inherits the properties and methods of the base class. This enables the reuse and extension of functions. Objects of the derived class are also instances of the base class and can use its methods.
class Mammal extends Animal {
function breathesAir() {
echo "inhale";
}
}
$horse = new Mammal;
$horse->setName("Louis");
phpWith PHP extend, the “Mammal” class inherits all the properties and methods of the “Animal” superclass. We can add a new function to “Mammal”, but we can also access inherited functions.
Examples for using PHP classes
PHP classes are very versatile. The following are practical examples of their use.
PHP instanceof
In PHP, instanceof is an operator to check whether an object belongs to a specific class or a class derived from it.
Here’s a simple example:
class Animal {
public function speak() {
echo "sound";
}
}
class Dog extends Animal {
public function speak() {
echo "bark";
}
}
$animal = new Dog();
if ($animal instanceof Animal) {
$animal->speak(); // Output: "bark"
}
phpFirst, we use instanceof to check whether $animal
is an instance of the PHP class “Animal” or one of its derived classes. Since $animal
is an instance of the derived class “Dog” (which inherits from Animal), the condition is fulfilled and the code in the If block is executed.
The instanceof
operator is useful if you want to ensure that you can access certain methods or properties without errors if the object does not have the expected class.
PHP classes and arrays
Classes can be stored in PHP arrays to design complex data structures.
class Product {
public $name;
public $price;
public function __construct($name, $price) {
$this->name = $name;
$this->price = $price;
}
}
$products = [
new Product("Laptop", 800),
new Product("Smartphone", 400),
new Product("Tablet", 300)
];
foreach ($products as $product) {
echo "Product: " . $product->name . ", Price: $" . $product->price . "<br>";
}
phpIn this example, we define the “Product” class to represent different products and store them in the “products” array. We can then loop through the array with PHP loops like the foreach construct to output the product names and prices.
PHP class constructor
The constructor is a special method within a PHP class that is automatically called when an object of this class is created. The purpose of a constructor is to initialize the initial states of an object or to perform other preparatory work required for the object to function correctly.
class Dog {
private $name;
private $color;
// Constructor
public function __construct($name, $color) {
$this->name = $name;
$this->color = $color;
}
public function sayHello() {
return "Hello, my name is {$this->name} and my color is {$this->color}.";
}
}
$dog = new Dog("Tom", "brown");
echo $dog->sayHello(); // Output: "Hello, my name is Tom and my color is brown."
phpCost-effective, scalable storage that integrates into your application scenarios. Protect your data with highly secure servers and individual access control.
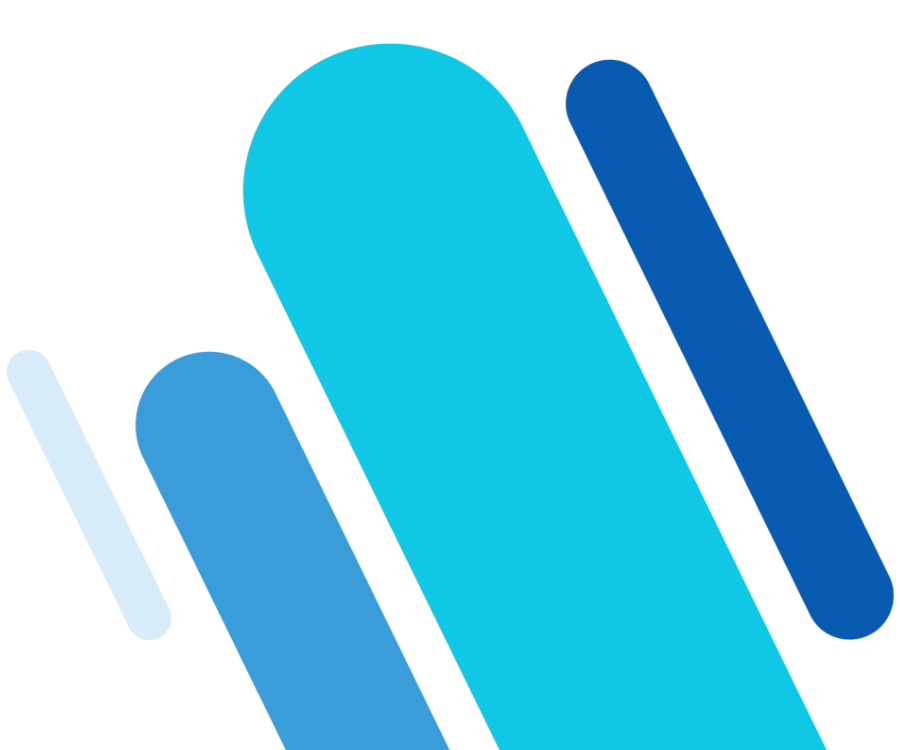