How to use Python arrays
Although there are no arrays in Python in the classical sense, you can use an elegant workaround by using the list function. With the help of various methods, you can easily serve your array in Python.
- 99.9% uptime
- PHP 8.3 with JIT compiler
- SSL, DDoS protection, and backups
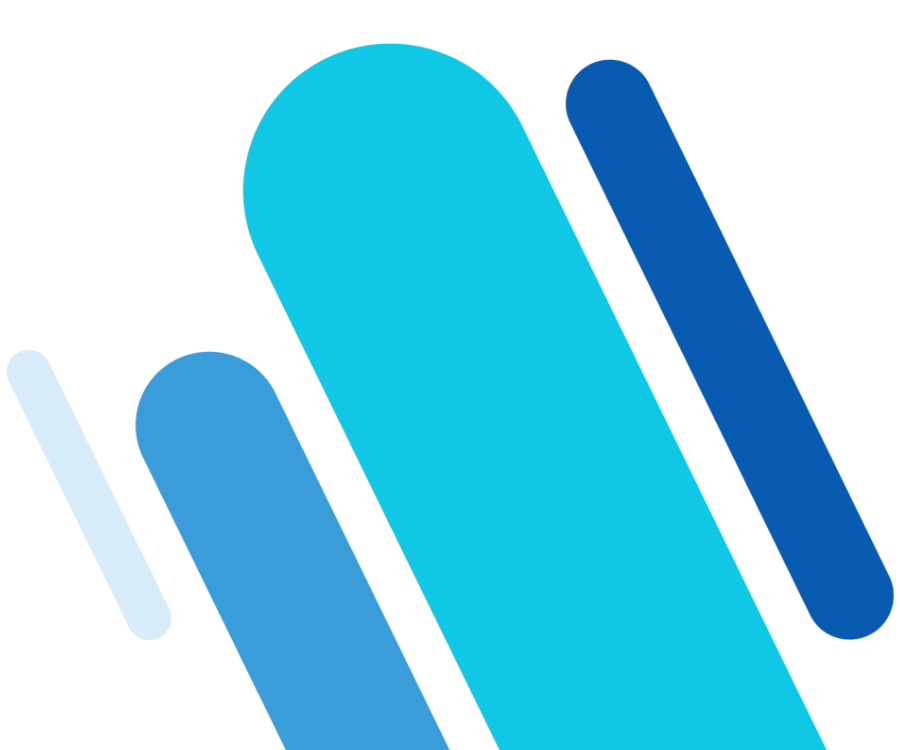
What are arrays and what are they used for?
Before we go into the meaning of arrays in Python, let’s clarify what arrays are in the first place and what they are typically used for. In Java, the term array refers to a data type or, more precisely, a container that holds a predetermined number of values of a certain type. The data type is not determined, the container can also contain objects or other arrays. However, the data type’s length and type must be defined in advance and cannot change your values afterwards. The process of storing the values inside the container is called initialization.
The classical arrays described above are actually not known in Python. An elegant way to get around this limitation is to use so-called lists in Python, which serve a similar function. Unlike containers in Java, lists in Python can contain different kinds of values.
Creating Arrays with Python Lists
A simple example of an array in Python is this:
# ingredients as individual variables
ingredient1 = "milk"
ingredient2 = "flour"
ingredient3 = "sugar"
# bundle ingredients in list
ingredients = [ingredient1, ingredient2, ingredient3]
In this case, you would have a list of ingredients, each of which you assign a fixed value (ingredient1, 2, 3, etc.). However, if this list grows many times longer, Python lets you filter a particular ingredient with an array. You can bundle as many values as you like and select them by the assigned number.
Accessing a specific element
To access a specific element, use the index number. Here is an example where you first query the value of the first item:
first = ingredients[0]
assert first == "milk"
Then in the second step you adjust this value:
ingredients[0] = "cream"
assert ingredients[0] == "cream"
The length of an array in Python
You must determine the length of an array in Python in advance, and you cannot change it afterwards. To set the length, select the highest value of the provided index numbers and increment it by 1. For the length of the array in Python, use the “len ( )” method. Here is an example:
number_ingredients = len(ingredients)
assert number_ingredients == 3
Adding elements to an array in Python
To add elements to your array in Python, it is best to use the “append ( )” method. Here’s how it works:
ingredients.append("salt")
assert ingredients[3] == "salt"
Delete elements from the array with pop ( ) or remove ( )
You have two ways to delete elements from an array in Python. The first method is “pop ( )”. If you want to remove the third ingredient (“milk”) from the array of ingredients introduced above, it works like this:
third = ingredients.pop(2)
assert third == "sugar"
assert len(ingredients) == 3
You have to remember that the counting starts at 0, so the first element gets the value 0, the second element gets the value 1 and so on.
The second method to remove, for example, the third ingredient from the array in Python is “remove ( )”. This looks like this:
ingredients.remove("cream")
assert "cream" not in ingredients
This removes the value “milk” from the array. However, it is important that this method deletes the corresponding value only on its first occurrence. If the value reappears later in the process, it is not automatically removed.
Looping elements in a Python array
You loop the elements of your array in Python using a “for in” loop, which you may already know from a Python tutorial. Use the following command to request the replay of each item in the Python array “ingredients”:
for ingredient in ingredients:
print (ingredient)
List of different methods for arrays in Python
While Python does not have arrays, it does allow lists instead, as explained above. For a quicker and more focused approach to these replacement arrays, it’s worth looking at the different methods Python provides, some of which we’ve already introduced. The following methods will help you learn internet programming language and work with arrays in Python:
Method | Description |
---|---|
append ( ) | Adds an element to the end of the list (see above). |
clear ( ) | This method deletes all elements from the list. |
copy ( ) | copy ( ) outputs a copy of the entire list. |
count ( ) | This method outputs the exact number of elements with a given value. |
extend ( ) | extend ( ) adds the entire list elements to the end of an array in Python. |
index ( ) | Outputs the index number of the first element with a given value. |
insert ( ) | Adds an element at a specified position. |
len ( ) | Use len ( ) to specify the length of an array in Python (see above). |
pop ( ) | Pop ( ) deletes an element at a specified position (see above). |
remove ( ) | remove ( ) removes the first element with a specified value. |
reverse ( ) | Use this method to reverse the order of the elements in your Python array. |
sort ( ) | With sort ( ) you can sort your list. |