How to use the Python list
Python lists help structure content and read it later. The various methods enable you to edit, sort, extend or shorten your list.
- DNS management
- Easy SSL admin
- API documentation
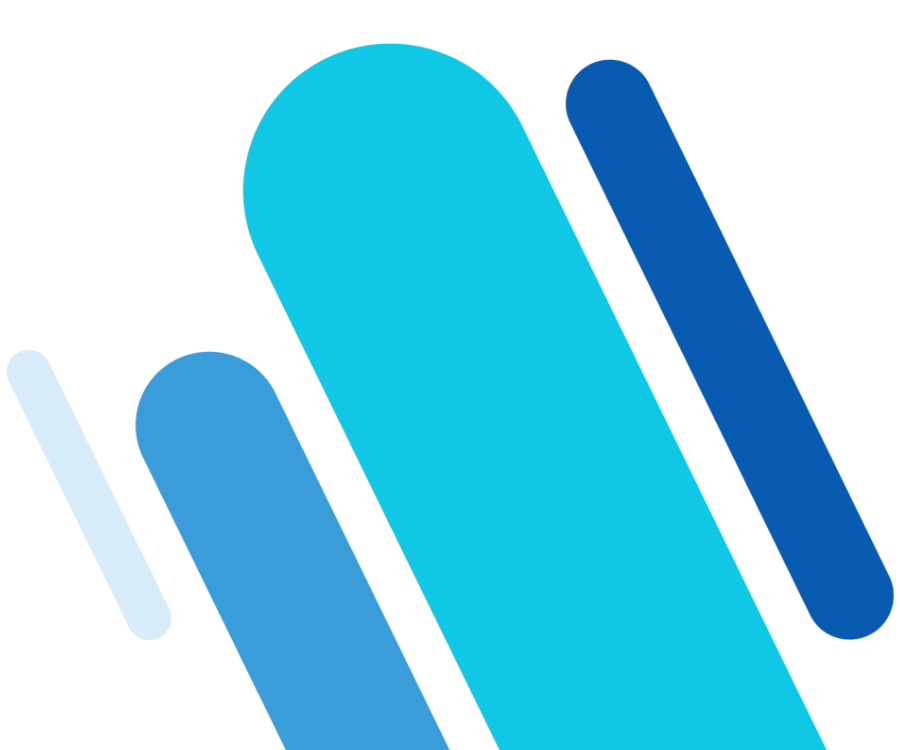
What are Python lists?
A Python list is useful if you want to store complex information clearly. It isn’t just simple data and object types that can be stored in a Python list, it is also possible to nest different values and lists. The Python list can be either homogeneous or heterogeneous. Homogeneous means that it only contains a single type of data. A heterogeneous structure on the other hand, involves different file types being concatenated in the list. A Python list’s versatility makes it an optimal alternative to an array. We’ve covered how this works in our Arrays in Python article.
How are Python lists constructed?
A Python list contains any number of values. These are enclosed by square braces and separated by commas in Python String.
cars = ['audi', 'ford', 'fiat', 'bentley']
Each value in the list is given an index so the Python list can be read again later. Filtering a specific value from the list requires writing the corresponding index number in square braces after the Python list. This will give the value you are looking for as an output. Remember that the internet programming language is always counted from 0. The content of the list may also be duplicated and divided by a Python Split.
first = cars[0]
assert first == 'audi'
Python list example
This simple example shown below will illustrate how to build a Python list and read it later. We’ll be using country names and putting them in square braces for this list:
countries = ["France", "Uruguay", "Germany", "Netherlands", "Ghana"]
If you want to read the countries from this Python list which aren’t in Europe, proceed with the following steps:
print(countries[1])
print(countries[4])
The output will be:
"Uruguay"
"Ghana"
Python sublists
A Python list can also contain sublists. These are like ordinary elements. These will look like:
countries_capitals = [
["France", "Uruguay", "Germany", "Ghana"],
["Paris", "Montevideo", "Berlin", "Accra"]
]
first_country = countries_capitals[0][0]
assert first_country == "France"
print(first_country)
The output will read:
"France"
Enter the following if you want to output the capital:
capitals = countries_capitals[1]
first_capital = capitals[0]
print (f"The first capital is {first_capital}.")
You will receive this as an output:
The first capital is Paris.
How do I extend Python lists?
There are three options available if you want to extend your Python list: Append, extend, and insert. We’ll will look into each of these methods.
With append
Use append to extend your list by one item:
countries = ["France", "Uruguay", "Germany", "Ghana"]
countries.append("Japan")
print(countries)
It should now read:
['France', 'Uruguay', 'Germany', 'Ghana', 'Japan']
With extend
Use extend to add multiple items to your Python list.
countries = ["France", "Uruguay", "Germany", "Ghana", "Japan"]
countries.extend(["Italy", "Canada", "Australia"])
print(countries)
This code results in:
['France', 'Uruguay', 'Germany', 'Ghana', 'Japan', 'Italy', 'Canada', 'Australia']
With insert
Insert allows you to insert a new element into the middle of your Python list. You must specify the new element and also assign an index number to it directly.
countries = ["France", "Uruguay", "Germany", "Ghana"]
countries.insert(1, "China")
assert countries[1] == "China"
print(countries)
This results in:
['France', 'China', 'Uruguay', 'Germany', 'Ghana']
Reminder: 'France' has the index number 0 and therefore remains first in the list.
How do I delete elements from a Python list?
You can use either remove or del to delete items from your Python list.
With remove
Remove allows you to remove an element by writing it in the corresponding braces. However, the element will only be deleted the first time if it occurs more than once.
countries = ["France", "Uruguay", "Germany", "Netherlands", "Ghana"]
countries.remove("Germany")
print(countries)
This produces the following output:
['France', 'Uruguay', 'Netherlands', 'Ghana']
With del
Alternatively, you can use del to remove an element from your Python list. You don’t need specify the element itself with this method, you can delete it using its index.
countries = ["France", "Uruguay", "Germany", "Netherlands", "Ghana"]
del countries[2]
assert "Germany" not in countries
print(countries)
The result is the same as the remove method.
How do I sort Python lists?
The sort function allows you to put your list in order with minimal effort. If you want to sort the countries from the list alphabetically, it works like this:
countries = ["France", "Uruguay", "Germany", "Netherlands", "Ghana"]
countries.sort()
print(countries)
It now reads:
['France', 'Germany', 'Ghana', 'Netherlands', 'Uruguay']
You can write this information in the empty braces to output a descending order:
countries = ["France", "Uruguay", "Germany", "Netherlands", "Ghana"]
countries.sort(reverse = True)
print(countries)
This will display the countries in the order "Uruguay" to "France".
Overview of methods
You can use the following methods for your Python list. You probably know some of them already from our Python tutorial.
Method | Description |
---|---|
Append | Adds an item to the end of your list. |
Clear | Removes all elements from the Python list. |
Copy | Outputs a copy of your list. |
Count | Counts all elements with a value in the Python list. |
Extend | Adds all entries to another list. |
Index | Searches for a specific element within the Python list and returns the index number. |
Insert | Inserts an element at a specified position. |
Pop | Removes an element from a specified position and uses this as the return value. |
Remove | Removes the first element with a specified value. |
Reverse | Reverses the Python list’s order. |
Sort | Sorts the list. |