How to send emails easily with PHPMailer
PHPMailer is a program library that regulates email transfer through PHP. PHPMailer is mainly used for contact forms on websites, but it also can be used to send private emails.
- The world’s leading email and calendar solution
- Secure hosting from a single source
- Anytime remote access with Microsoft Outlook
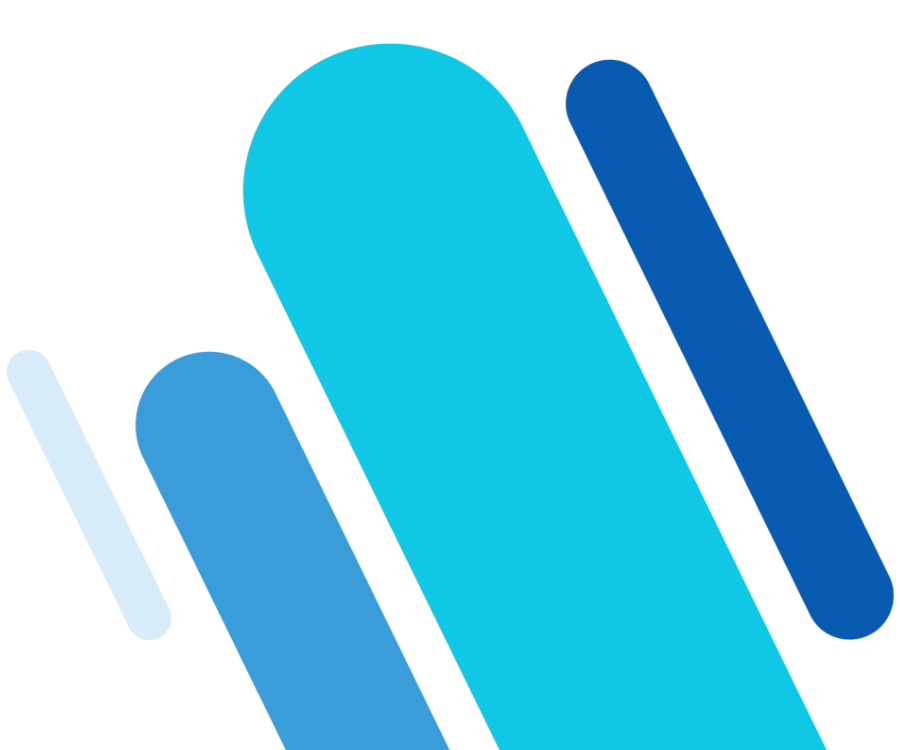
What is PHPMailer?
PHPMailer is a mail extension for PHP, which is developed and maintained by the PHP community. Emails sent using PHPMailer are less likely to end up in a spam folder than those sent with the built-in mail function from PHP. This is because mails that are written with PHPMailer are sent using SMTP. SMTP stands for Simple Mail Transfer Protocol and is used to transfer emails over the internet. In addition, PHPMailer also supports HTML mails and attachments, unlike the built-in mail function.
What is PHPMailer used for?
PHPMailer is a bit more tedious to use when compared to other email providers or integrated mail programs on your computer, which might leave you wondering why people choose to use it. The PHP framework simplifies creating automated responses, such as those needed for a web store. Additionally, PHPMailer is also suitable for contact forms on websites.
What are the requirements for PHPMailer?
You need an SMTP server to use PHPMailer. It’s up to you whether you use a mail server from a provider or set up your own server. It’s best to use Composer (a package manager for PHP) to install the PHP extension.
Mastering the basics of PHP is another important requirement for using PHPMailer. Our PHP tutorial for beginners can help you if you are having trouble with the basics of PHP.
How to install PHPMailer step by step
Step 1: Download current version of PHPMailer
Download the latest version of PHPMailer. You can do this with Composer or manually from GitHub.
Option 1: Download using Composer
If you have installed the package manager Composer,you can simply use this command:
composer require phpmailer/phpmailer
bashImportant: If you installed PHPMailer with Composer, you must include Composer in your PHP code in order to send mails.
The following line of code can be used:
require_once "vendor/autoload.php";
bashThe keyword “require_once” ensures that Composer is only included once. Otherwise, you may encounter program errors. The installation using Composer is now complete.
Option 2: Download directly from GitHub
The PHPMailer source files can also be downloaded manually by clicking on the Code button in the corresponding GitHub repository and downloading the ZIP file. If Git is installed on your system, you can alternatively clone the repository using a command-line command.
Step 2: Unzip files
If you downloaded PHPMailer’s source code manually, you have to open the ZIP files. Select the location where you want to install PHPMailer and include PHPMailer in your script. Assuming you have unpacked the PHPMailer files in a folder called PHPMailer, you can use the following lines of code:
use PHPMailer\PHPMailer\PHPMailer;
use PHPMailer\PHPMailer\Exception;
/*Class for handling exceptions and errors*/
require 'C:/PHPMailer/src/Exception.php';
/*PHPMailer class*/
require 'C:/PHPMailer/src/PHPMailer.php';
/*SMTP class needed to connect to an SMTP server*/.
require 'C:/PHPMailer/src/SMTP.php';
/*When creating a PHPMailer object, pass the parameter “true” to activate exceptions (messages in the event of an error)*/
$email = new PHPMailer(true);
phpHow to send emails with PHPMailer step by step
Step 1: Include namespaces
Ensure the namespaces are correct in order to access PHPMailer. Use
statements are required for this, so your code should contain the following lines:
<?php
use PHPMailer\PHPMailer\PHPMailer;
use PHPMailer\PHPMailer\SMTP;
use PHPMailer\PHPMailer\Exception;
phpYou can access the individual classes by including the names after the last slash.
Step 2: Catch errors
While PHPMailer is a very reliable way to send emails, errors can occur from time to time. Including a try catch statement in an email dispatch will prevent sensitive data on your mail server from being sent to users as an error message:
try {
// Try to create a new instance of PHPMailer class, where exceptions are enabled
$mail = new PHPMailer (true);
// (...)
} catch (Exception $e) {
echo "Mailer Error: ".$e->getMessage();
}
phpStep 3: Authenticate with SMTP
You must authenticate yourself with SMTP to use PHPMailer. Enter the address of your mail server next to the appropriate protocol (either TLS/SSL or SMTP) and include the port with your username and password. The protocol and port you use depend on your mail provider. The respective server data can be retrieved from the mail provider’s website.
$mail->isSMTP();
$mail->SMTPAuth = true;
// Personal data
$mail->host = "smtp.domain.com";
$mail->Port = 587;
$mail->username = "name.surname@domain.com";
$mail->password = "testpassword4321";
$mail->SMTPSecure = PHPMailer::ENCRYPTION_STARTTLS;
phpIn place of ENCRYPTION_STARTTLS
, you can also use ENCRYPTION_SMTPS
as the encryption method. With ENCRYPTION_SMTPS
, a connection is only established if TLS TLS is active. This means that communication with a server is only possible if the server supports the encryption measures required for secure transmission. With StartTLS,the connection to the mail server is established without encryption. Only afterward is encryption applied. If it’s not possible to apply TLS encryption, the remaining data exchange will occur unencrypted.
SMTPS offers a higher level of security, while StartTLS hasbetter compatibility. Because of its compatibility, StartTLS is generally the preferred choice.
Step 4: State the recipient of the email
Now you can designate the recipients of your email in the script.
// Sender
$mail->setFrom('info@example.com', 'name');
// Recipient, the name can also be stated
$mail->addAddress('info@example.com', 'name');
// Copy
$mail->addCC('info@example.com');
// Blind copy
$mail->addBCC('info@example.com', 'name');
phpStep 5: Add the contents of the mail
Don’t forget the contents of your email. This usually consists of a subject and a text, which can be specified as both HTML and non-HTML versions. It’s important to note that older software may not be able to handle the current HTML5 standard, so it may be a good idea to create your email using HTML.
You can also easily send attachments with PHPMailer using the addAttachment function. Images, music, documents, videos and GIFs can also be sent with PHPMailer. You can also rename your attachments with an optional second parameter which is transferred to the function.
$mail->isHTML(true);
// Subject
$mail->Subject = 'The subject of your mail';
// HTML content
$mail->Body = 'The mail text as HTML content. <b>bold</b> elements are allowed.';
$mail->AltBody = 'The text as a simple text element';
// Add attachment
$mail-> addAttachment("/home/user/Desktop/sampleimage.png", "sampleimage.png");
phpStep 6: Use the correct character encoding
It’s a good idea to enable UTF-8 in PHPMailer to prevent display errors from occurring when processingaccented letters from other languages, especially when using different attachments. You can do this by adding the following lines of code to your PHP script:
$mail->CharSet = 'UTF-8';
$mail->Encoding = 'base64';
phpStep 7: Send email
Now, it’s time to send your email. Use the following command to do this:
$mail->send();
phpIt’s best to place all of the code shown in the send request in the try statement block of your script so you can catch any errors.
Code example for sending an email with PHPMailer
Below is all the code you need to send an email with containing an image attachment to a recipient of your choice using PHPMailer:
<?php
use PHPMailer\PHPMailer\PHPMailer;
use PHPMailer\PHPMailer\SMTP;
use PHPMailer\PHPMailer\Exception;
require_once "vendor/autoload.php";
// Enable or disable exceptions via variable
$debug = true;
try {
// Create instance of PHPMailer class
$mail = new PHPMailer($debug);
if ($debug) {
// issue a detailed log
$mail->SMTPDebug = SMTP::DEBUG_SERVER;
}
// Authentication with SMTP
$mail-> isSMTP();
$mail->SMTPAuth = true;
// Login
$mail->Host = "smtp.domain.com";
$mail->Port = 587;
$mail->Username = "name.surname@domain.com";
$mail->Password = "testpassword4321";
$mail->SMTPSecure = PHPMailer::ENCRYPTION_STARTTLS;
$mail->setFrom('info@example.com', 'name');
$mail->addAddress('info@example.com', 'name');
$mail-> addAttachment("/home/user/Desktop/sampleimage.png", "sampleimage.png");
$mail->CharSet = 'UTF-8';
$mail->Encoding = 'base64';
$mail->isHTML(true);
$mail->Subject = 'The subject of your mail';
$mail->Body = 'The mail text in HTML content. <b>bold</b> elements are allowed.';
$mail->AltBody = 'The text as a simple text element';
$mail->send();
} catch (Exception $e) {
echo "Message could not be sent. Mailer Error: ".$e->getMessage();
}
php- Personalized email address
- Access your emails from anywhere
- Highest security standards
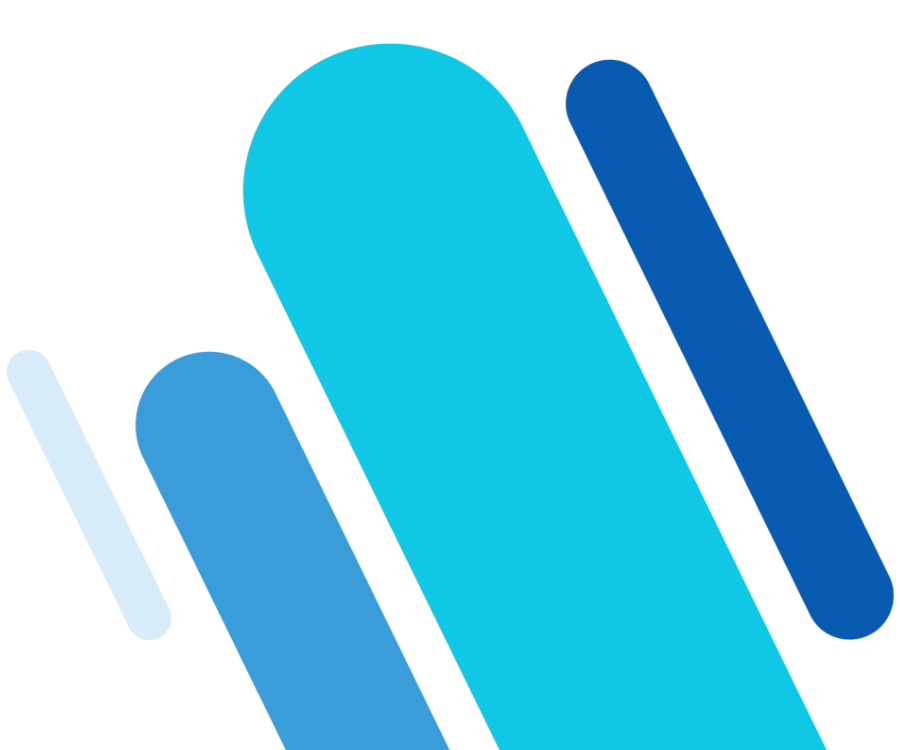