How to use PHP if-else for conditional logic and programming
PHP if-else gives you control over the flow of your code. You can specify which statements are to be executed under which conditions, allowing you to control your program more precisely.
What is PHP if-else?
PHP if-else is a basic control structure that allows you to make conditional decisions in a program. It checks a specific condition or a condition nested with PHP operators and executes a block of code if it evaluates to true. If the condition is false, an alternative code block is selected. This allows you to define behaviors for different scenarios. These can be, for example, calling PHP functions or arithmetic calculations.
What the syntax of PHP if-else looks like
The syntax of a simple if-else statement in PHP is as follows:
if (condition) {
// Code to be executed if the condition is true.
} else {
// Code to be executed if the condition is false.
}
php- if (condition): The condition to be checked is specified here. If this condition is true, the code in the first block (after the opening curly bracket) is executed.
- { … }: These curly brackets contain the code block that is called if the condition is true.
- else: This part is optional. If the condition in the If part is false, the code in the Else block is selected.
Discover Deploy Now from IONOS to run your development projects on a stable hosting platform. Rapid integration with your GitHub repository enables efficient deployment of your code updates, without much hassle. With Deploy Now, you can carry out your development work securely and conveniently.
What is elseif?
The elseif statement is an extension of PHP if-else. It’s used to evaluate several conditions. Each is assigned its own code block:
if (condition1) {
// Code that is executed if condition1 is true
} elseif (condition2) {
// Code that is executed if condition2 is true
} else {
// Code that is executed if none of the conditions is true}
phpThere is an alternative, more compact notation for PHP if-elseif without curly brackets:
$var = 5;
if ($var > 5):
echo "var is greater than 5";
elseif ($var == 5):
echo "var is 5";
else:
echo "var is smaller than 5";
endif;
phpIn this version, you must end the If statement with endif
.
Find out more about PHP programming in our PHP tutorial. We also recommend that you take a look at the comparisons of PHP vs. Python and PHP vs. JavaScript to learn about the advantages and disadvantages of each language.
- DNS management
- Easy SSL admin
- API documentation
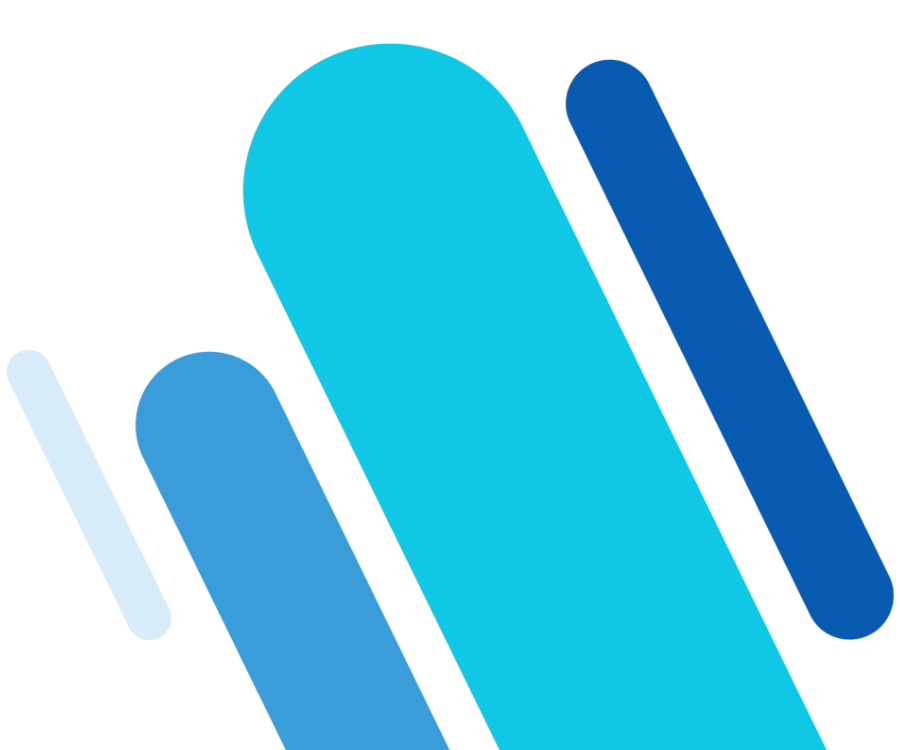
Examples for using if-else in PHP
PHP if-else statements can be used flexibly and in different forms for applications.
PHP if-else shorthand
The PHP if-else shorthand allows you to represent a simple conditional statement in a single line. It’s often referred to as a Ternary Operator because it has three parts: the condition, the value that is returned if the condition is true, and another value if it’s false.
$age = 20;
$status = ($age >= 18) ? "adult" : "minor";
phpIn this example, we check whether the variable $age
is greater than or equal to 18. Since it’s greater, the value “adult” is assigned to the variable $status
.
Conditional logic for database queries
When retrieving information from a MySQL database using PHP, you can convert the data into instances of PHP classes and use conditional logic:
class user {
public $name;
public $age;
public function __construct($name, $age) {
$this->name = $name;
$this->age = $age;
}
public function isadult () {
if ($this->age >= 18) {
return true;
} else {
return false;
}
}
}
phpFirst, we define the Class User with the properties “name” and “age” and the method “is adult()”.
$userlist = array();
while ($row = mysqli_fetch_assoc($result)) {
$user = new user($row['name'], $row['age']);
$userlist[] = $user;
if ($user->isadult ()) {
echo $user->name . "is adult.<br>";
} else {
echo $user->name . " is a minor.<br>";
}
}
phpWe declare an empty array variable $userList
to store user data. With PHP loops like while
we can iterate through the result records. In the while
loop, we create an object of class “user” for each user record and add it to the $userList
. Finally, we use PHP if-else to check whether the user is of legal age or not and output a corresponding message.
Cost-effective, scalable storage that integrates into your application scenarios. Protect your data with highly secure servers and individual access control.
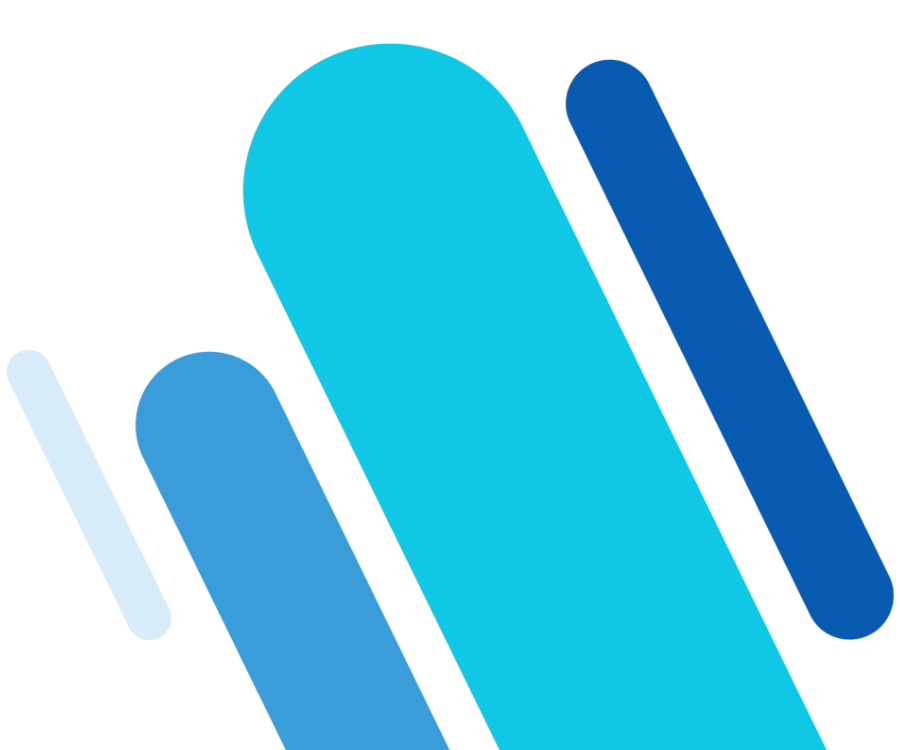