How to store and process data with PHP variables
PHP variables let you store, transform, and manipulate data. For example, you can drop strings, filter and sort arrays, or perform complex calculations.
What are PHP variables?
A PHP variable is a type of container that can store information while a PHP program is running for later access. Variables are identified by a name and can contain values from different data types, like numbers, strings, booleans, or complex data structures like arrays or objects. Variables are often elementary components of PHP functions and PHP classes. They’re used to collect user input, store intermediate results, personalize content in web applications, or manage session data. You can also use PHP to retrieve information from a MySQL database and store and process the entries in variables.
With Deploy Now from IONOS you’re in control of the development process. Choose your Git repository and use automatic framework detection with GitHub Actions to deploy your application. With Deploy Now, you’ll get custom domains, SSL security, and log-based visitor statistics.
The syntax of PHP variables
In PHP, variables are created by prefixing the variable name with a dollar sign ($) and then assigning a value to it. Here’s the basic syntax:
$var = value;
phpThe rules for PHP variables are:
-
Variable name: A variable name in PHP must start with a dollar sign ($) followed by letters, numbers, or underscores. The first letter after the dollar sign must not be a number. For example:
$MyVariable
,$value_1
, but not$1variable
. -
Case sensitive: PHP is case sensitive.
$myVariable
and$MyVariable
are considered different variables. - Reserved words: Avoid PHP reserved words as variable names. For example, “echo”, “if”, “while”, and “foreach” are reserved words.
- Special characters: Variable names must not contain special characters (except for the underscore) or spaces.
If you want to learn more about PHP variables and their functions, we recommend checking our PHP tutorial from our guide. We’ve also done comparison pieces on PHP vs. Python and PHP vs. JavaScript.
- DNS management
- Easy SSL admin
- API documentation
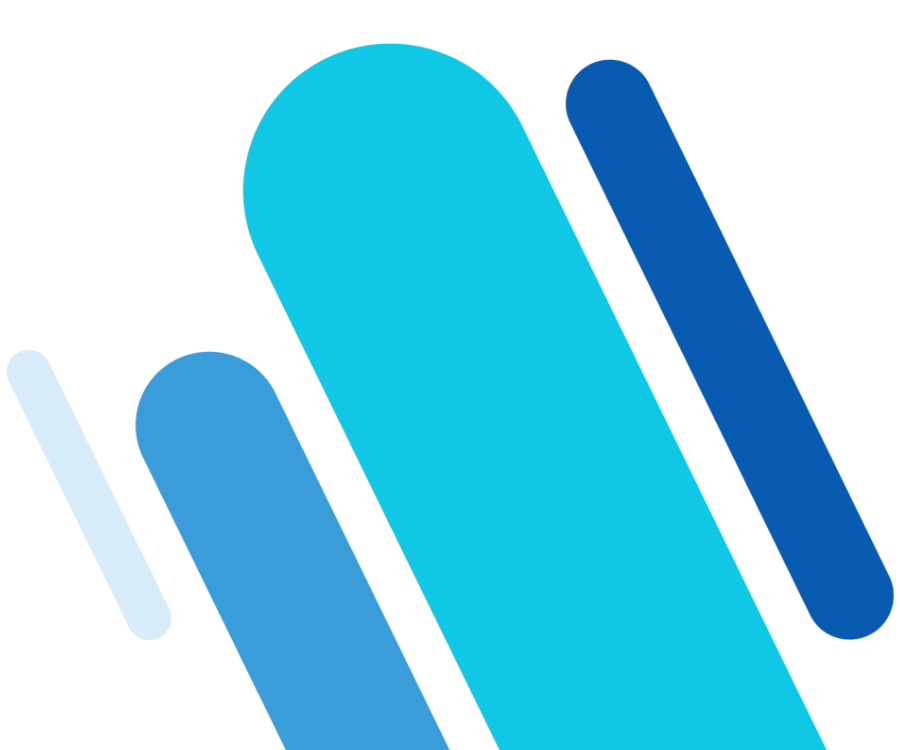
What types of PHP variables are there?
PHP is a weakly typed language, meaning you don’t need to specify the PHP variable type explicitly. The data type is automatically detected, depending on the assigned value. This lets you flexibly implement variables in PHP. Here are some of the most important data types:
- Integer (int): This type of data is used to represent integers without decimals.
- Float (float): Floats, also known as floating point numbers, are numbers with decimal places.
- String (string): Strings refer to strings of text.
- Boolean (bool): Booleans represent truth values and can be either true or false.
- Array (array): An array is an ordered list of values that can be stored under a single name. Arrays can contain values of different data types.
- Object (object): An object is an instance of a class and can store methods and properties (variables).
- NULL: NULL is a special value that indicates that a variable has no value. As a result, the PHP variable is defined, i.e. it has been defined with a value of NULL.
Examples using PHP variables
PHP variables have many uses. Here’s how you can output, link, and use variables globally.
Echo PHP variable
PHP echo is a simple way to display variables and their contents on the screen.
$var = "blue";
echo $var;
phpThe output will be:
blue
phpConcatenate PHP variables
You can link PHP variables with PHP operators like the concatenation operator .
to strings.
$var = "blue";
echo "The sky is " . $var
phpOn the screen you see:
The sky is blue
phpPHP variable global
In PHP, you can create and initialize global variables using the $GLOBALS supervariable.
$GLOBALS['myVar'] = "This is a global variable";
phpThis assignment makes the variable $myVar
global and can be accessed from any part of your PHP code.
Cost-effective, scalable storage that integrates into your application scenarios. Protect your data with highly secure servers and individual access control.
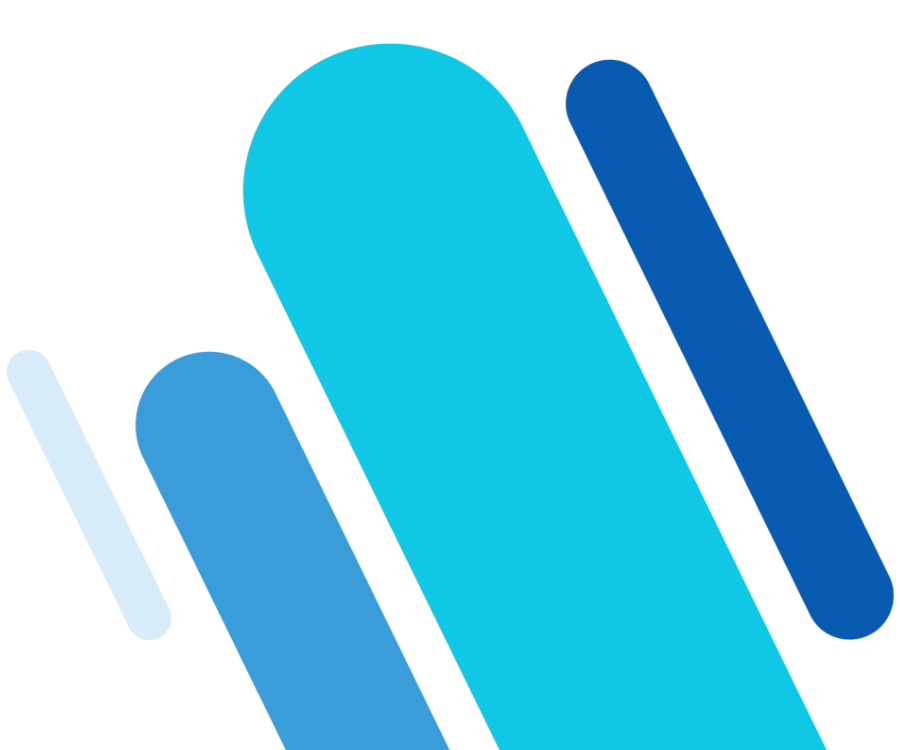